Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Beginner's Guide to Rust: Get Started with Rust 2021 Edition

Rust is a modern systems programming language that focuses on safety, speed, and concurrency. It was first introduced in 2010 by Mozilla Research and has gained significant popularity over the years. With its unique features and syntax, Rust offers a powerful alternative to languages like C++ and Java. In this comprehensive guide, we will walk you through the basics of Rust and help you get started with the latest 2021 edition.
Why Rust?
Before we jump into learning Rust, let's understand why it has become an attractive choice for developers. Rust eliminates entire categories of bugs that are common in traditional programming languages. Its innovative ownership system and strict compile-time checks ensure memory safety, thread safety, and prevent common programming mistakes. With Rust, you can confidently build high-performance applications with minimal errors and crashes.
Setting Up Rust
To begin your Rust journey, you need to set up the development environment. Rust has excellent support for all major operating systems, including Windows, macOS, and Linux. Simply head to the official Rust website and download the appropriate installer for your system. The installation process is straightforward and well-documented. Once installed, you can verify your Rust installation by running a simple command in the terminal.
4.2 out of 5
Language | : | English |
File size | : | 820 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 544 pages |
Hello, World! in Rust
Now that you have Rust up and running, let's dive into some code. Every programming language starts with the classic "Hello, World!" example, and Rust is no exception. Open your favorite code editor and create a new file with a ".rs" extension. Rust files usually have the ".rs" extension, short for Rust source code. In your file, write the following code:
fn main(){println!("Hello, World!"); }
Save the file and open the terminal in the same directory. Use the "cd" command to navigate to the directory and run the following command to compile and execute the code:
$ rustc filename.rs $ ./filename
Congratulations! You have just written and executed your first Rust program. It may seem simple, but it lays the foundation for further learning and exploration.
Variables and Data Types
Like any programming language, Rust has a wide range of data types to work with. From integers and floats to strings and booleans, Rust offers all the essential types for building robust applications. Let's explore some common data types and how to work with them in Rust.
Integer types in Rust include i8
, i16
, i32
, i64
, u8
, u16
, u32
, and u64
. The "i" stands for "signed," indicating that the type can hold both positive and negative values. Similarly, the "u" stands for "unsigned," representing only positive values.
To declare a variable in Rust, you can use the let
keyword. For example, to declare an integer variable named "x" with the value of 10, you can write:
let x: i32 = 10;
Strings, another important data type, can be declared and manipulated using the String
class. Rust provides many useful methods for working with strings, such as concatenation, slicing, and length calculation.
These are just a few examples of data types in Rust. Throughout your learning journey, you will encounter many more data types and learn how to use them effectively for different purposes.
Control Flow and Conditionals
Control flow is a fundamental concept in programming that allows you to make decisions and execute different blocks of code based on certain conditions. Rust provides several control flow constructs, including if-else statements, loops, and match expressions.
The if-else statement works similarly to other programming languages. Let's say we have a variable x
, and we want to check if it's greater than 10. Based on the result, we can print different messages. Here's how it looks in Rust:
let x = 15; if x > 10 { println!("x is greater than 10"); }else { println!("x is less than or equal to 10"); }
Loops in Rust include while
and for
. The while
loop executes a block of code repeatedly until a given condition becomes false. The for
loop, on the other hand, iterates over a collection, such as an array or a range of numbers.
Match expressions in Rust provide a powerful way to handle multiple cases and choose the appropriate action based on the value. It is often used to handle enums and pattern matching. Match expressions ensure exhaustive handling, preventing any runtime errors or unexpected behaviors.
Functions and Modules
Functions are the building blocks of any programming language. They allow you to encapsulate reusable pieces of code and execute them whenever needed. Rust supports the creation of both named and anonymous functions.
fn greet(name: &str){println!("Hello, {}!", name); }fn main(){greet("John"); greet("Jane"); }
Modules, on the other hand, provide a way to organize your code into logical units. They help break down large codebases into smaller, manageable components. Rust's module system ensures code encapsulation, clear boundaries, and easy code reuse.
Error Handling in Rust
One of Rust's standout features is its robust error handling mechanism. Rust imposes a strict error handling discipline to prevent runtime errors and crashes. It achieves this using the famous Result
type and the Option
type.
The Result
type represents the result of an operation that can succeed or fail. It can hold either an Ok
value or an Err
value. By explicitly propagating errors, Rust enforces error handling at each step, ensuring reliability and security.
The Option
type, similar to Result
, represents a value that can be present or absent. It helps prevent null pointer exceptions and encourages safe programming practices.
Concurrency and Parallelism
Rust's fearless concurrency model enables you to write efficient, concurrent code with ease. It provides several abstractions, such as threads, channels, and atomic operations, to handle concurrent tasks without compromising safety.
Rust's ownership system ensures that concurrent access to shared data is handled correctly and prevents race conditions and data races. It enforces strict rules during compile-time, highlighting potential issues before they manifest in runtime bugs.
In this beginner's guide, we have only scratched the surface of Rust's capabilities. Rust offers a vast ecosystem of libraries, frameworks, and tools that can help you build anything from command-line utilities to web applications and embedded systems. By mastering Rust, you can unlock a new world of opportunities and become part of a vibrant community of developers. So, don't hesitate; start your Rust journey today!
4.2 out of 5
Language | : | English |
File size | : | 820 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 544 pages |
Learn to program with Rust 2021 Edition, in an easy, step-by-step manner on Unix, the Linux shell, macOS, and the Windows command line. As you read this book, you’ll build on the knowledge you gained in previous chapters and see what Rust has to offer.
Beginning Rust starts with the basics of Rust, including how to name objects, control execution flow, and handle primitive types. You’ll see how to do arithmetic, allocate memory, use iterators, and handle input/output. Once you have mastered these core skills, you’ll work on handling errors and using the object-oriented features of Rust to build robust Rust applications in no time.
Only a basic knowledge of programming in C or C++ and familiarity with a command console are required. After reading this book, you’ll be ready to build simple Rust applications.
What You Will Learn
- Get started programming with Rust
- Understand heterogeneous data structures and data sequences
- Define functions, generic functions, structs, and more
- Work with closures, changeable strings, ranges and slices
Use traits and learn about lifetimes
Who This Book Is For
Those who are new to Rust and who have at least some prior experience with programming in general: some C/C++ is recommended particularly.
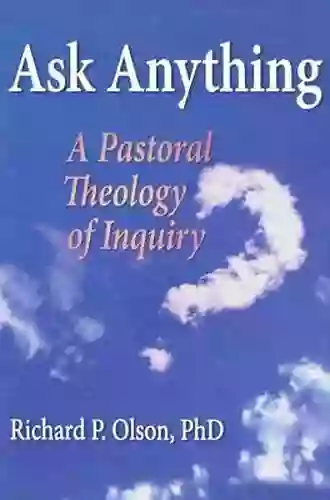

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
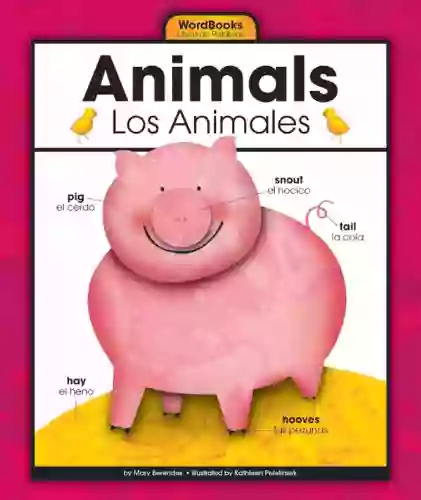

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
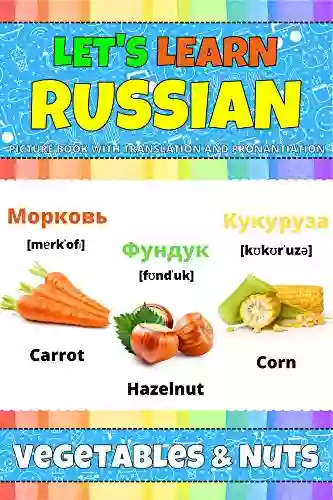

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
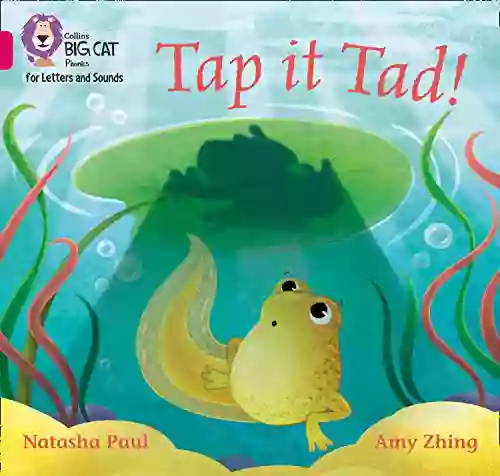

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
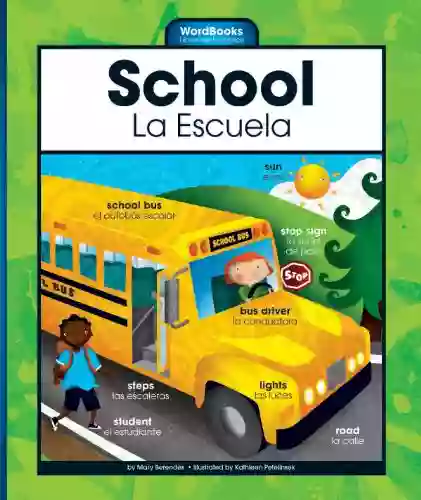

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
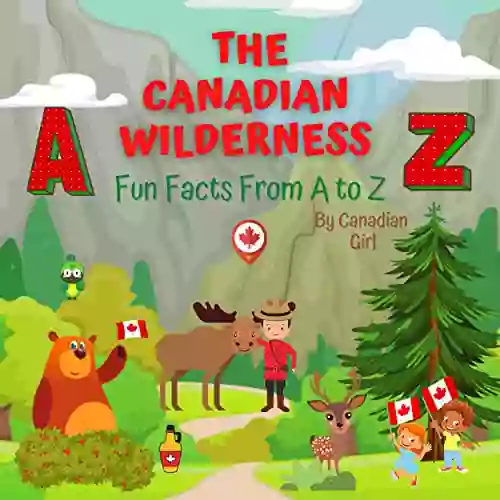

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
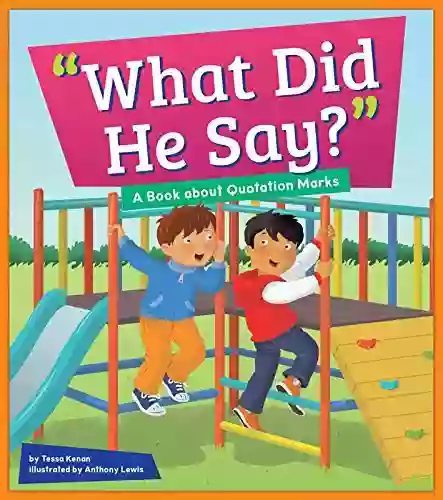

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
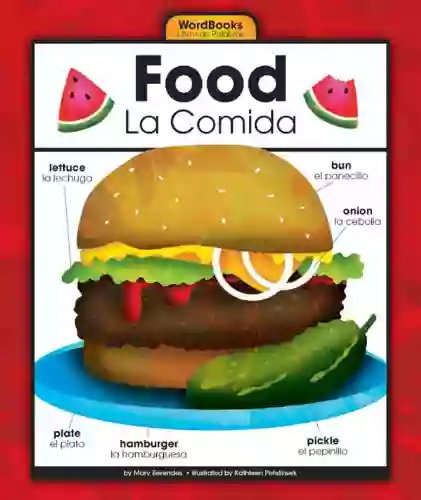

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
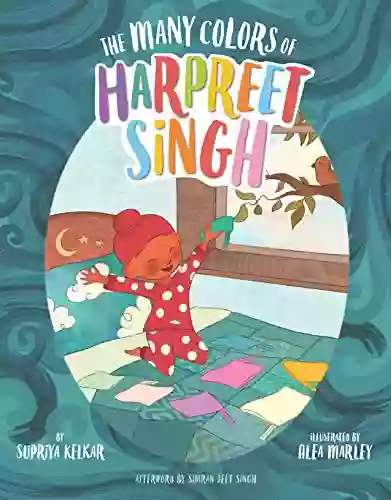

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
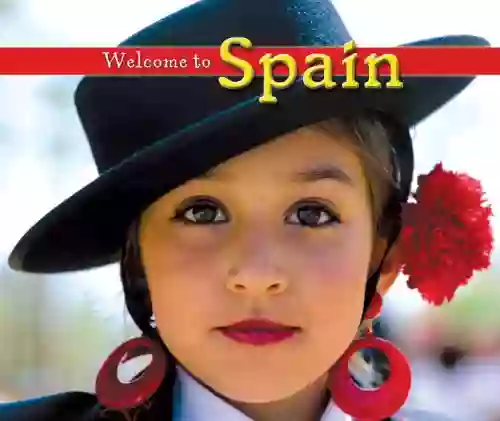

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
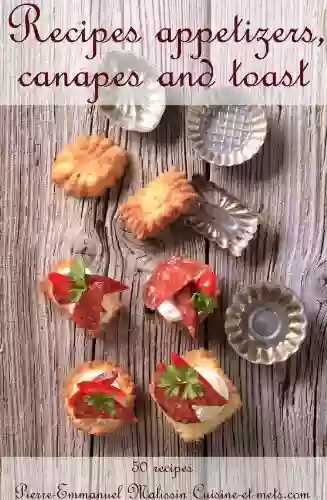

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
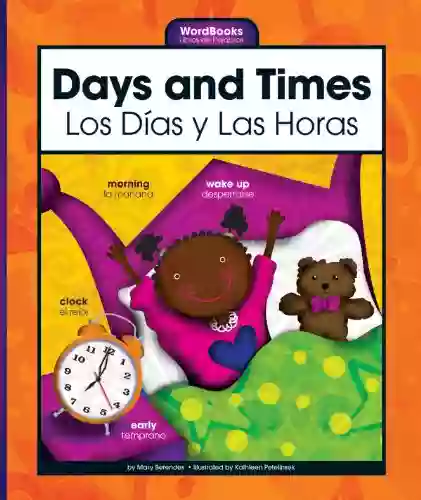

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!


- Isaiah PowellFollow ·17.1k
- Greg CoxFollow ·6.7k
- Russell MitchellFollow ·19.8k
- Ronald SimmonsFollow ·12.4k
- Theo CoxFollow ·3.2k
- Eli BrooksFollow ·2.2k
- E.M. ForsterFollow ·12.1k
- Shawn ReedFollow ·15.7k