Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Learn How To Implement The Gang Of Four Design Patterns Using Swift Improve

The Gang of Four (GoF) design patterns are an essential part of software development. Originally defined by four authors, Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, in their book "Design Patterns: Elements of Reusable Object-Oriented Software," these patterns provide proven solutions to common design problems in software development.
Swift, Apple's powerful programming language, has gained immense popularity among developers for its simplicity, expressiveness, and safety. By utilizing Swift's features, you can easily implement GoF design patterns to improve your code structure and maintainability.
In this article, we will explore some of the popular GoF design patterns and learn how to implement them using Swift. By understanding these patterns and being able to apply them effectively, you will enhance your software development skills and produce more efficient and maintainable code.
4.3 out of 5
Language | : | English |
File size | : | 1633 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 277 pages |
Lending | : | Enabled |
1. Singleton Pattern
The Singleton pattern ensures that only one instance of a class exists throughout the application's lifecycle. This pattern is extremely useful when you want to restrict the instantiation of a class to a single object. It is often used for creating shared resources or managing global states.
To implement the Singleton pattern in Swift, you can utilize the language's static properties and lazy initialization feature. Here's an example:
class Singleton { static let shared = Singleton() private init() {}}
By making the initializer private and providing a static property to access the shared instance, you ensure that only one instance of the class can be created.
2. Factory Method Pattern
The Factory Method pattern provides an interface for creating objects, but allows subclasses to decide which class to instantiate. It is useful when you want to decouple the object creation logic from the client code.
In Swift, you can implement the Factory Method pattern using a combination of protocols and inheritance. Here's an example:
protocol Shape { func draw() }class Circle: Shape { func draw(){print("Drawing a circle") }}class Square: Shape { func draw(){print("Drawing a square") }}class ShapeFactory { func createShape() -> Shape { fatalError("You must override this method") }}class CircleFactory: ShapeFactory { override func createShape() -> Shape { return Circle() }}class SquareFactory: ShapeFactory { override func createShape() -> Shape { return Square() }}
In this example, the `ShapeFactory` class acts as the base factory that defines the common interface for creating shapes. Subclasses such as `CircleFactory` and `SquareFactory` inherit from the base factory and provide the specific implementation for creating their respective shapes.
3. Observer Pattern
The Observer pattern defines a one-to-many dependency between objects, where a change in one object's state triggers updates in multiple other objects. It is useful when you want to establish a loosely coupled relationship between subjects and observers.
In Swift, you can implement the Observer pattern using a combination of protocols, delegates, and closures. Here's an example:
protocol Observer: AnyObject { func update() }class Subject { private var observers = [Observer]() func addObserver(_ observer: Observer){observers.append(observer) }func notifyObservers(){observers.forEach { observer in observer.update() }}}class ConcreteObserver: Observer { func update(){print("Observer notified of a change") }}let subject = Subject() let observer = ConcreteObserver() subject.addObserver(observer) subject.notifyObservers()
In this example, the `Subject` class maintains a list of observers and provides methods for adding observers and notifying them of changes. The `ConcreteObserver` class implements the `update` method from the `Observer` protocol, defining the specific action to be taken when notified.
4. Prototype Pattern
The Prototype pattern allows you to create new objects by copying existing ones, without relying on their concrete classes. It is useful when creating new objects is expensive or complex.
In Swift, you can implement the Prototype pattern by utilizing Swift's `NSCopying` protocol. Here's an example:
class Shape: NSCopying { var name: String init(name: String){self.name = name }func copy(with zone: NSZone? = nil) -> Any { return Shape(name: name) }}let originalShape = Shape(name: "Circle") let clonedShape = originalShape.copy() as! Shape print("Original shape: \(originalShape.name)") print("Cloned shape: \(clonedShape.name)")
In this example, the `Shape` class adopts the `NSCopying` protocol and provides an implementation for the `copy(with zone:)` method. By calling the `copy` method on an existing object, you can create a new object with the same properties.
By learning and implementing the Gang of Four design patterns using Swift, you can significantly improve your code structure, maintainability, and productivity as a software developer. These patterns provide valuable solutions to common design problems and can be applied to various software development scenarios.
In this article, we explored four popular design patterns: Singleton, Factory Method, Observer, and Prototype. Each pattern showcased how they can be implemented using Swift's unique features and syntax.
As you continue your journey in software development, don't overlook the importance of design patterns. They serve as a powerful toolset that can empower you to write cleaner, more maintainable, and efficient code.
4.3 out of 5
Language | : | English |
File size | : | 1633 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 277 pages |
Lending | : | Enabled |
Software developers need to solve various problems. Many times, these problems are the same or similar to the ones they’ve already encountered in other projects.
Wouldn’t it be great to apply the solution you’ve found instead of reinventing the wheel over and over again?
That’s precisely the reason why software design patterns exist. A design pattern is a standardized way to address a recurring problem. Relying on a proven strategy will not only save you time, but you can rest assured that it’s indeed the right choice.
Design patterns are the result of a long evolution process. It all started with a book published in 1994 - yes, it’s that old! - called “Design Patterns - Elements of Reusable Object-Oriented Software.” That’s a quite tedious title, so we usually refer to it as “the book by the gang of four.” The gang consists of four renowned software engineers: Erich Gamma, Ralph Johnson, Richard Helm, and John Vlissides. They identified the most significant common issues that occurred in multiple projects and developed best practices to solve them.
The best part: these solutions are (programming) language-agnostic. You can use the design patterns with any object-oriented programming language.
Many modern programming languages and frameworks have integrated the GoF patterns. You don’t have to write additional code to support say the Iterator or the Observer.
Swift is no exception: it provides many advanced language features and constructs such as type extensions, lazy initialization, and predefined protocols that let us adopt and integrate the design patterns into our projects easily.
This book covers all these topics and teaches best practices you can apply in your upcoming projects.
We’ll talk about the benefits of understanding and applying the design patterns, the value they provide, and also about their limitations.
Then, we delve into the creational design patterns:
- the Singleton,
- the Prototype,
- the Factory Method,
- the Builder,
- and the Abstract Factory design pattern
We’re going to take a closer look at the structural design patterns. We discuss:
- the Adapter,
- the Decorator,
- the Façade,
- the Flyweight,
- and the Proxy pattern
In the final part of this book, we discuss the behavioral design patterns:
- the Chain of Responsibility,
- the Iterator,
- the Observer,
- and we finish with the State design pattern
For each design pattern, we discuss the following:
- When to use the specific design pattern?
- How can it be implemented using Swift 5?
- What are the challenges and pitfalls of using the given pattern?
Throughout the book, I provide coding examples that can be applied in real-world situations.
Károly Nyisztor is a veteran software engineer and instructor.
He has worked with large companies such as Apple, Siemens, and SAP. Károly has designed and built several enterprise frameworks, and he holds twelve patents related to inventions in the field of mobile computing.
After 18 years, he left the corporate world to start his own business.Â
Since 2016, he's fully committed to teaching. As an instructor, he aims to share his 20+ years of software development expertise. Károly teaches:
- Software Architecture,
- Object-Oriented Programming and Design
- Swift and iOS Programming,
- and other, programming-related topics
You can find Károly Nyisztor's courses and books on all major platforms including Amazon, Lynda, LinkedIn Learning, Pluralsight, Udemy, and iTunes.
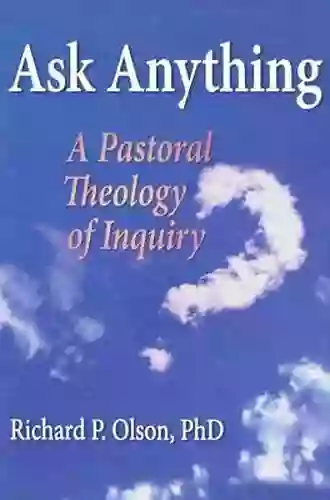

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
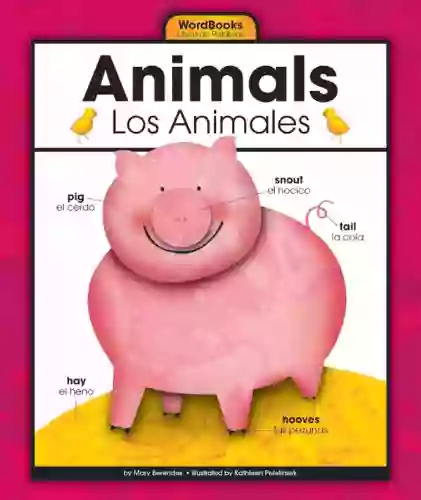

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
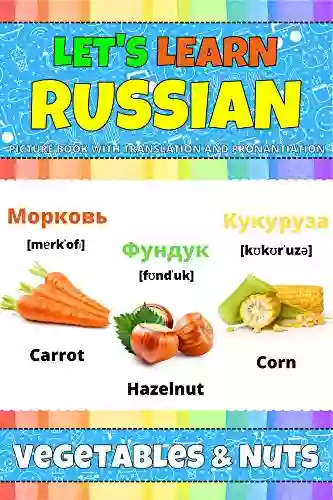

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
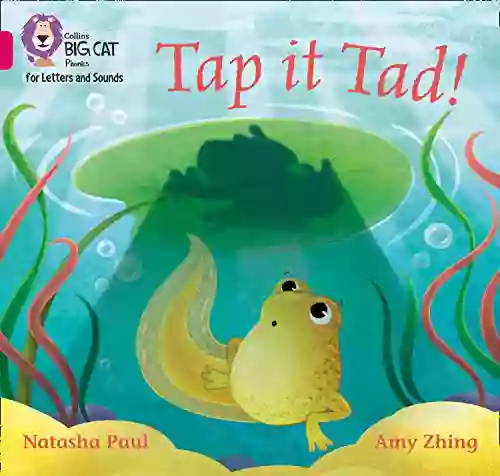

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
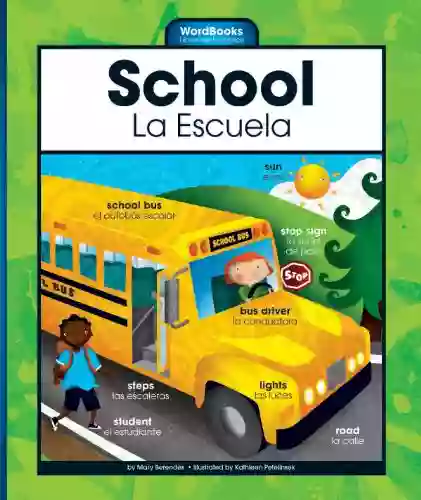

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
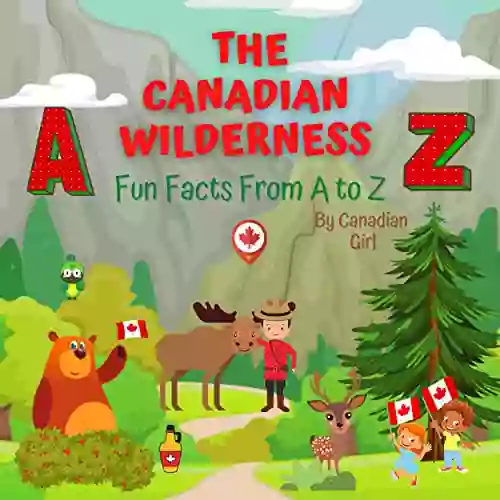

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
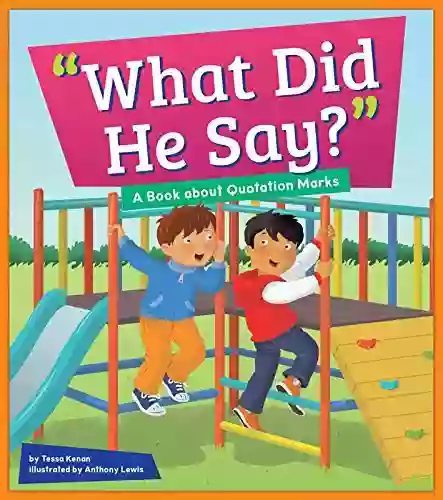

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
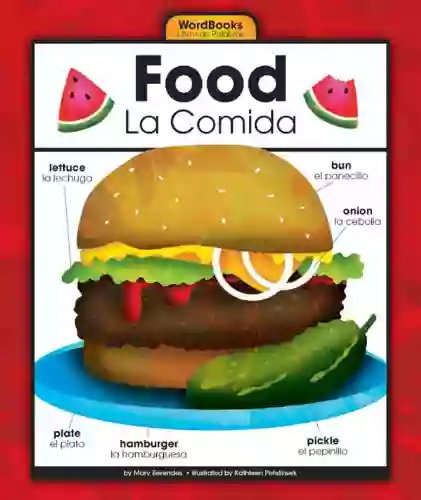

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
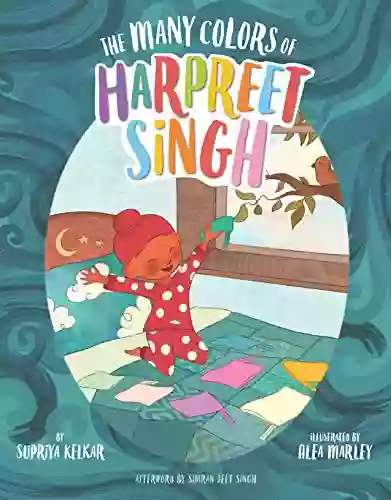

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
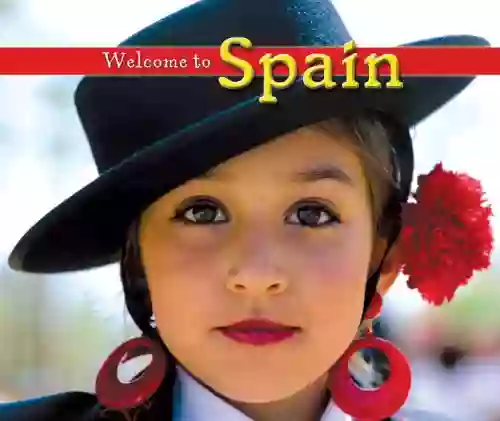

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
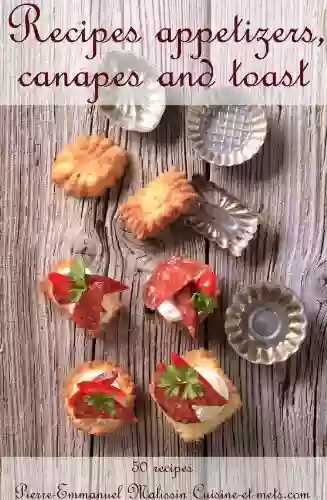

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
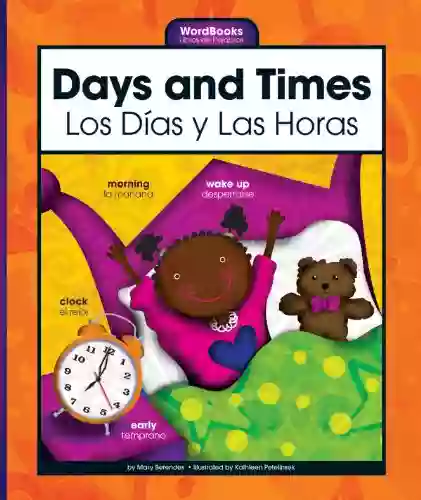

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!








- Alex ReedFollow ·7.3k
- Dawson ReedFollow ·14k
- Julian PowellFollow ·3.5k
- Thomas MannFollow ·4.2k
- John GrishamFollow ·19.8k
- Edwin CoxFollow ·18.9k
- Wade CoxFollow ·4.3k
- William WordsworthFollow ·16.6k