Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
How To Improve Your Javascript Programs Using Functional Techniques

Are you looking to take your JavaScript programming skills to the next level? Functional programming techniques might be just what you need. By adopting a functional approach, you can improve the quality and simplicity of your code, making it easier to maintain and debug.
What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. In functional programming, functions are treated as first-class citizens, meaning they can be assigned to variables and passed as arguments to other functions.
JavaScript is a versatile language that supports functional programming alongside other paradigms such as object-oriented programming. By leveraging functional techniques, you can make your code more concise, modular, and reusable, leading to significant improvements in code quality and maintainability.
4.2 out of 5
Language | : | English |
File size | : | 6641 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 272 pages |
The Benefits of Functional Programming in JavaScript
There are several advantages to using functional programming techniques in JavaScript:
- Modularity: Functional programming promotes modularity by breaking down complex problems into smaller, more manageable functions. This allows you to tackle one piece of the puzzle at a time, leading to cleaner and more maintainable code.
- Reusability: Functional programming encourages the creation of pure functions that produce the same output given the same input. This makes your code more reusable, as functions can be easily plugged into different parts of your program without causing unexpected side effects.
- Testability: Functional programming makes it easier to test your code since pure functions don't depend on external state or mutable data. Tests can be written to ensure that given a specific input, the function always produces the expected output.
- Conciseness: Functional programming reduces boilerplate code by relying on higher-order functions and composition. This leads to more expressive and concise code, making it easier to understand and maintain.
- Parallelism: By avoiding state mutations, functional programming enables easy parallelization of computations. Pure functions can be executed independently, allowing for efficient use of multiple cores and improving performance.
Functional Techniques to Improve Your JavaScript Programs
Now that you understand the benefits of functional programming, let's dive into some techniques you can apply to improve your JavaScript programs:
1. Pure Functions
Pure functions are the building blocks of functional programming. They always return the same output given the same input and don't cause any side effects. By using pure functions, you can isolate pieces of logic and make your code more testable and reusable.
For example, consider the following impure function:
function greet(name){console.log(`Hello, ${name}!`); }
This function not only prints a greeting but also writes to the console, which is a side effect. To make it pure, we can modify it as follows:
function greet(name){return `Hello, ${name}!`; }
Now the function returns the greeting without causing any side effects. It can be easily tested and reused in different parts of the program.
2. Higher-Order Functions
Higher-order functions are functions that take one or more functions as arguments or return another function. They enable function composition and provide a powerful way to abstract common patterns in your code.
Consider the following example where we have an array of numbers and want to filter out the even ones:
const numbers = [1, 2, 3, 4, 5, 6];
function isEven(number){return number % 2 === 0; }
const evenNumbers = numbers.filter(isEven);
By using a higher-order function like `filter`, we avoid writing a loop and conditionals to achieve the same result. Higher-order functions make your code more expressive and declarative.
3. Function Composition
Function composition is the process of combining two or more functions to produce a new function. It allows you to create complex behavior by chaining simple functions together.
Here's an example where we have two functions, `double` and `square`, and want to apply them to a number:
function double(number){return number * 2; }
function square(number){return number * number; }
const result = square(double(5));
By composing the `double` and `square` functions, we can easily apply both operations to a number. Function composition promotes code reuse and improves readability by breaking down complex tasks into smaller, reusable functions.
4. Immutable Data Structures
Immutable data structures are data structures that cannot be modified after they are created. Instead of modifying existing data, you create new data structures whenever changes are needed.
In JavaScript, objects and arrays are mutable by default. However, several libraries like Immutable.js and Immer provide immutable data structures that can help enforce immutability in your code.
Using immutable data structures prevents accidental mutations, making your code more predictable and easier to reason about. It also enables efficient change detection, which is crucial for performance optimization.
Functional programming techniques offer numerous benefits for improving your JavaScript programs. By embracing concepts like pure functions, higher-order functions, function composition, and immutable data structures, you can write code that is more modular, reusable, testable, and maintainable.
While functional programming might require a shift in mindset and some initial learning, the benefits far outweigh the investment. Give it a try, and you'll likely find that your JavaScript programs become cleaner, more concise, and more enjoyable to work with.
4.2 out of 5
Language | : | English |
File size | : | 6641 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 272 pages |
Summary
Functional Programming in JavaScript teaches JavaScript developers functional techniques that will improve extensibility, modularity, reusability, testability, and performance. Through concrete examples and jargon-free explanations, this book teaches you how to apply functional programming to real-life development tasks
Purchase of the print book includes a free eBook in PDF, Kindle, and ePub formats from Manning Publications.
About the Technology
In complex web applications, the low-level details of your JavaScript code can obscure the workings of the system as a whole. As a coding style, functional programming (FP) promotes loosely coupled relationships among the components of your application, making the big picture easier to design, communicate, and maintain.
About the Book
Functional Programming in JavaScript teaches you techniques to improve your web applications - their extensibility, modularity, reusability, and testability, as well as their performance. This easy-to-read book uses concrete examples and clear explanations to show you how to use functional programming in real life. If you're new to functional programming, you'll appreciate this guide's many insightful comparisons to imperative or object-oriented programming that help you understand functional design. By the end, you'll think about application design in a fresh new way, and you may even grow to appreciate monads!
What's Inside
- High-value FP techniques for real-world uses
- Using FP where it makes the most sense
- Separating the logic of your system from implementation details
- FP-style error handling, testing, and debugging
- All code samples use JavaScript ES6 (ES 2015)
About the Reader
Written for developers with a solid grasp of JavaScript fundamentals and web application design.
About the AuthorLuis Atencio is a software engineer and architect building enterprise applications in Java, PHP, and JavaScript.
Table of Contents
PART 1 THINK FUNCTIONALLY
- Becoming functional
- Higher-order JavaScript
PART 2 GET FUNCTIONAL
- Few data structures, many operations
- Toward modular, reusable code
- Design patterns against complexity
PART 3 ENHANCING YOUR FUNCTIONAL SKILLS
- Bulletproofing your code
- Functional optimizations
- Managing asynchronous events and data
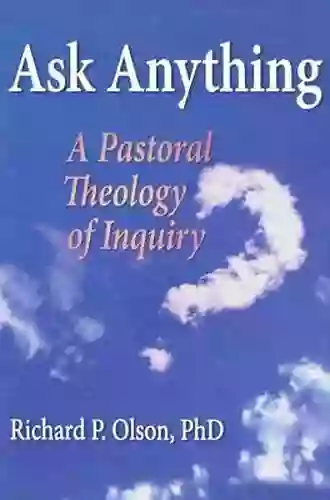

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
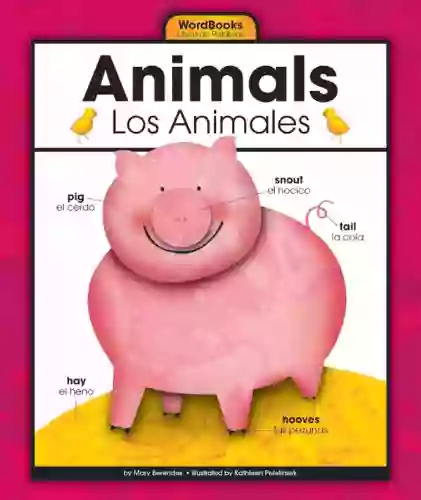

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
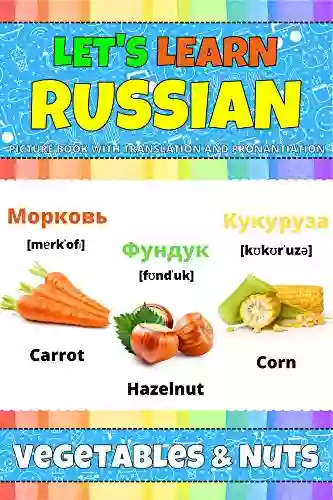

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
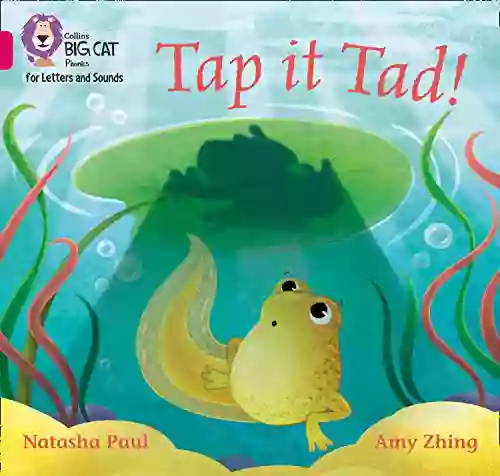

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
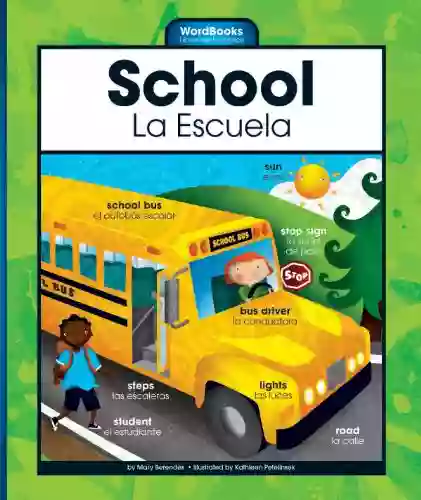

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
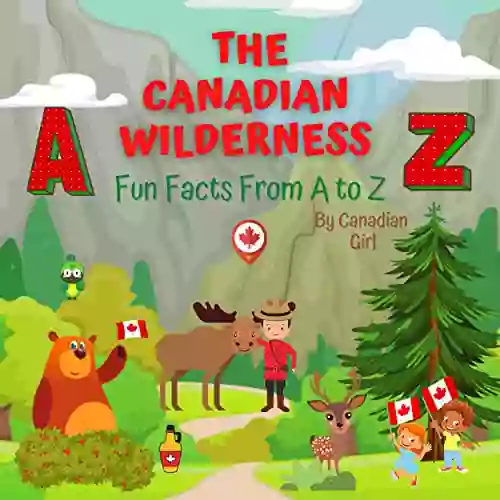

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
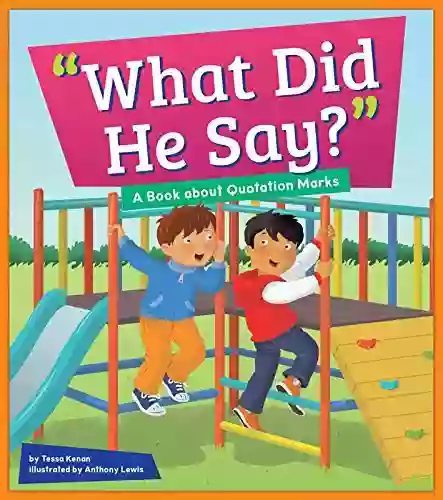

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
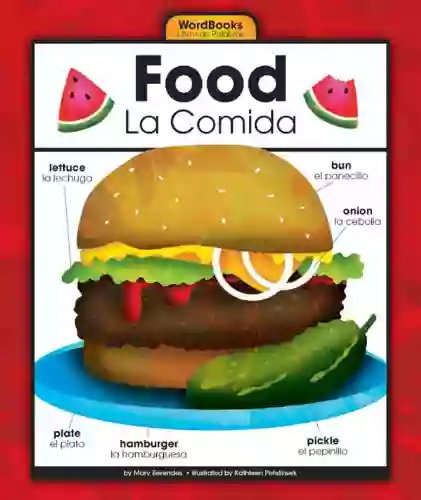

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
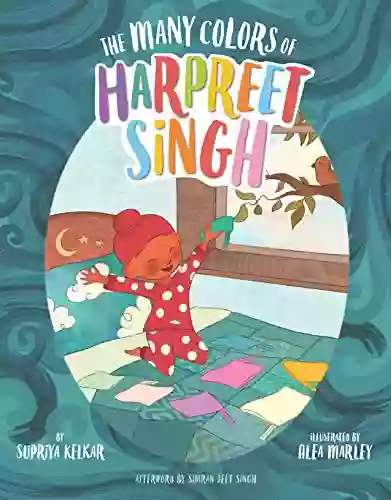

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
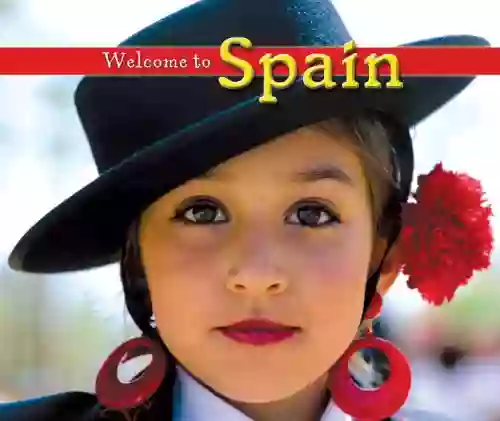

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
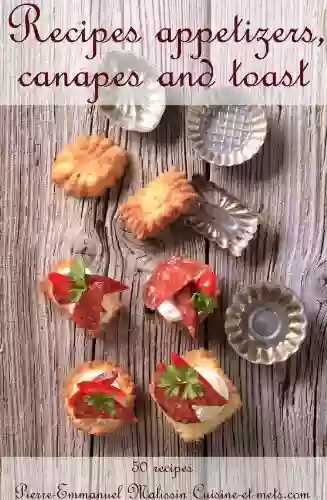

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
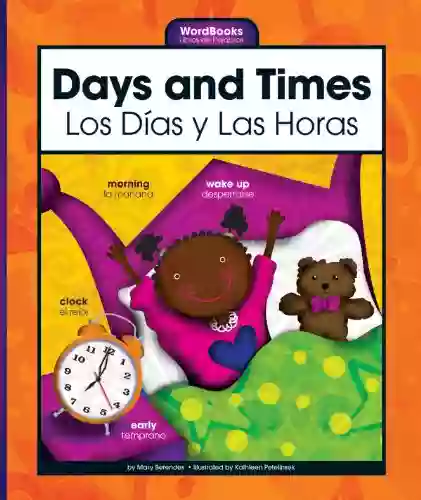

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
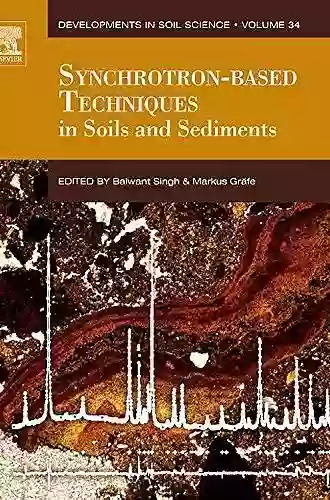



- Rodney ParkerFollow ·16.2k
- Zadie SmithFollow ·7.3k
- Eddie PowellFollow ·2.9k
- Joe SimmonsFollow ·2.6k
- Ron BlairFollow ·4.7k
- Duane KellyFollow ·11.5k
- Neil GaimanFollow ·18.6k
- Jaden CoxFollow ·11.5k