Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
A Lightweight Introduction To The Hibernate Framework

Are you a developer looking for an efficient way to work with relational databases in your Java applications? Look no further! In this article, we will delve into the world of Hibernate, a powerful object-relational mapping framework that simplifies database access and management. Whether you are a beginner or an experienced developer, understanding Hibernate will take your database interactions to the next level.
Why Choose Hibernate?
Hibernate provides a convenient and intuitive approach to working with databases in Java. It maps Java objects to database tables and handles the underlying SQL queries, enabling you to focus on the business logic of your application without getting bogged down by intricate database operations. Hibernate takes care of the tedious and error-prone tasks, such as connection management, transaction handling, and data retrieval, giving you more time to focus on delivering a superior user experience.
Getting Started
Before we dive into the technical details, let's understand the basic concepts behind Hibernate. At its core, Hibernate is an Object-Relational Mapping (ORM) framework. It allows you to define a mapping between your Java classes and the database tables they correspond to. This mapping is described using XML configuration files or annotations, making it easy to maintain and modify as your application evolves.
4.1 out of 5
Language | : | English |
File size | : | 405 KB |
Text-to-Speech | : | Enabled |
Enhanced typesetting | : | Enabled |
Print length | : | 193 pages |
Screen Reader | : | Supported |
When you use Hibernate, you work with Java objects instead of writing complex SQL queries. Hibernate takes care of transforming your object-oriented code into SQL, ensuring seamless integration between your application and the database. This abstraction layer simplifies the development process, improves code readability, and enhances maintainability.
The Hibernate Configuration
One of the first steps in using Hibernate is configuring your application. The Hibernate configuration file, often named hibernate.cfg.xml, contains important settings such as database connection details, dialect, and mapping files. With this configuration in place, Hibernate knows how to establish a connection to your database and communicate with it.
Let's take a quick look at a sample Hibernate configuration file:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/mydatabase</property> <property name="connection.username">myuser</property> <property name="connection.password">mypassword</property> <!-- Add more properties as needed --> </session-factory> </hibernate-configuration>
In this configuration, we define the database driver class, URL, username, and password. You can customize other properties according to your specific project needs. Once the configuration is set up, we can move on to creating our Java classes and their corresponding mapping files.
Mapping Our Java Classes
In Hibernate, each Java class that corresponds to a database table is referred to as an Entity. We define these entities using either XML mapping files or annotations, depending on our preference. The mapping file or annotation specifies the database table name, column names, primary key, and relationships with other entities.
Here's an example of a simple Java class annotated with Hibernate annotations:
@Table(name = "employees") @Entity public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @Column(name = "age") private int age; }
In this example, we define an Employee entity that maps to the "employees" table in the database. The @Table annotation specifies the table name, while the @Entity annotation marks this class as an entity.
The @Id and @GeneratedValue annotations indicate that the "id" field is the primary key, and its value will be automatically generated upon insertion. Other fields are mapped to their respective columns using the @Column annotation.
Working with Hibernate Sessions
Now that we have our configuration set up and entities defined, we can start utilizing Hibernate's session management capabilities. A session represents a single unit of work with the database; it encapsulates the database connection and provides methods for saving, updating, and querying entities.
The session is obtained using a session factory, which is responsible for creating, managing, and pooling database connections. The session factory is typically built during the application startup phase, and it is thread-safe and can be reused throughout your application.
Here's an example of how to obtain a session and perform database operations:
Session session = sessionFactory.openSession(); Employee employee = new Employee(); employee.setName("John Doe"); employee.setAge(30); Transaction transaction = session.beginTransaction(); session.save(employee); transaction.commit(); session.close();
In this code snippet, we open a session using the session factory and create a new instance of the Employee entity. We then begin a transaction, save the employee object to the database, and commit the transaction. Finally, we close the session to release the database connection.
Querying the Database
One of the primary benefits of Hibernate is its powerful querying capabilities. Hibernate provides a rich set of query options, including HQL (Hibernate Query Language),criteria queries, and native SQL queries. These enable you to fetch data from the database with ease and flexibility.
Here's an example of using HQL to retrieve all employees with an age greater than 25:
Query<Employee> query = session.createQuery("FROM Employee WHERE age > 25", Employee.class); List<Employee> employees = query.getResultList();
In this query, we use the HQL syntax to specify the selection criteria. The query.getResultList() method executes the query and returns a list of Employee objects that match the criteria.
Congratulations! You have now been introduced to the fundamentals of the Hibernate framework. We covered the benefits of using Hibernate, the important configuration steps, mapping Java classes to database tables, working with Hibernate sessions, and querying the database. Armed with this knowledge, you can start leveraging Hibernate's power to build robust and efficient Java applications that interact seamlessly with relational databases.
Remember, Hibernate is a vast framework with many advanced features and concepts. This article serves as a lightweight to get you started on your Hibernate journey. As you delve deeper into the framework, you will discover more techniques, best practices, and optimizations that will take your skills to the next level. Happy coding!
4.1 out of 5
Language | : | English |
File size | : | 405 KB |
Text-to-Speech | : | Enabled |
Enhanced typesetting | : | Enabled |
Print length | : | 193 pages |
Screen Reader | : | Supported |
If you’re looking for a short, sweet, and simple (or re) to Hibernate, this is the book you want. Through clear real-world examples, you’ll learn Hibernate and object-relational mapping from the ground up, starting with the basics. Then you’ll dive into the framework’s moving parts to understand how they work in action.
Storing Java objects in relational databases is usually a challenging and complex task for any Java developer, experienced or not. This book, like others in the Just series, delivers a concise, example-driven tutorial for Java beginners. You’ll gain enough knowledge and confidence to start working on real-world projects with Hibernate.
- Compare how JDBC and Hibernate work with object persistence
- Learn how annotations are used to create Hibernate applications
- Understand how to persist and retrieve Java data structures
- Focus on the fundamentals of associations and their mappings
- Delve into advanced concepts such as caching, inheritance, and types
- Walk through the Hibernate Query Language API, with examples
- Develop Java Persistence API applications, using Hibernate as the provider
- Work hands-on with code snippets to understand the technology
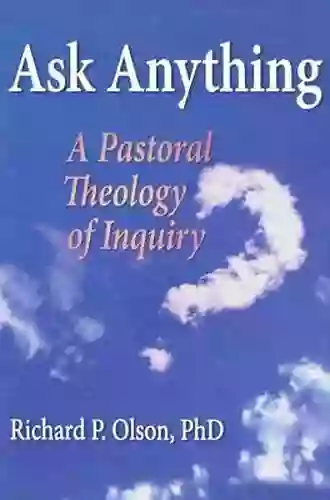

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
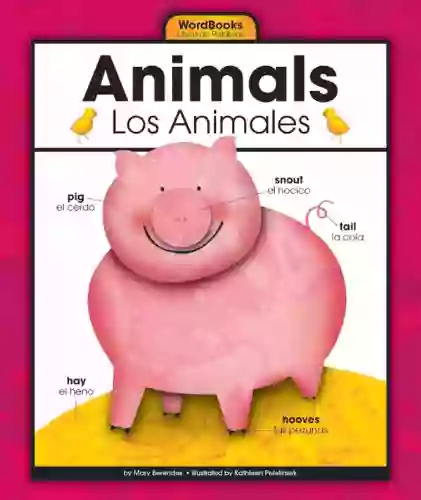

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
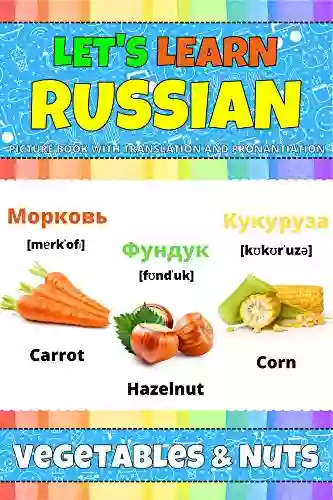

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
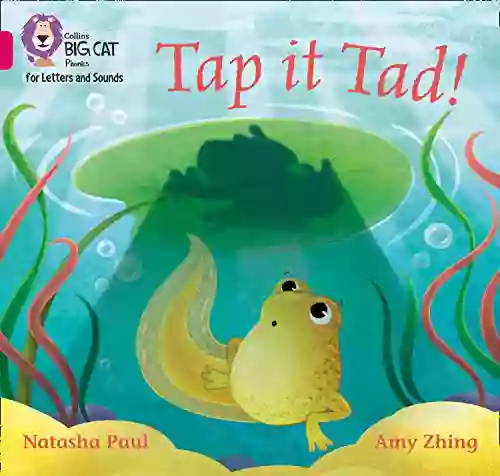

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
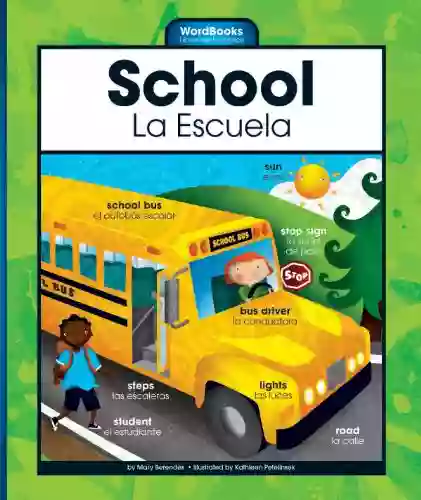

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
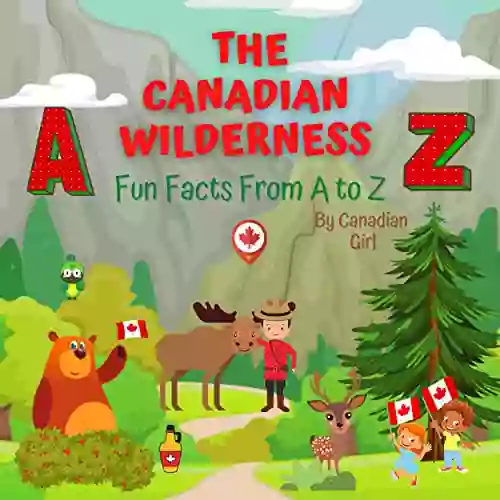

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
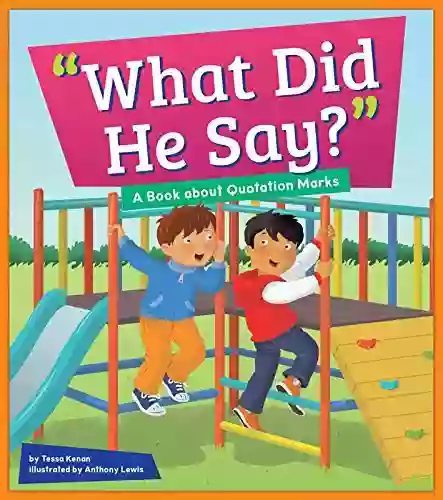

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
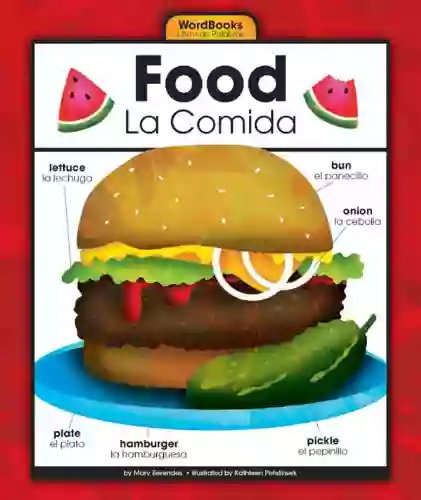

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
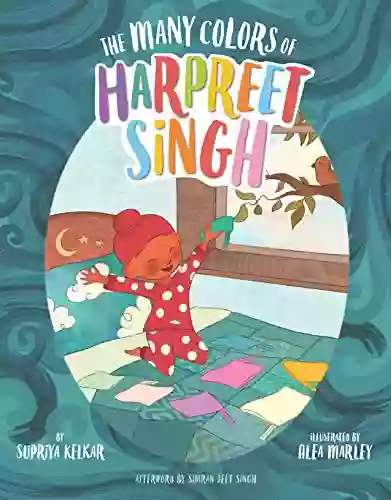

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
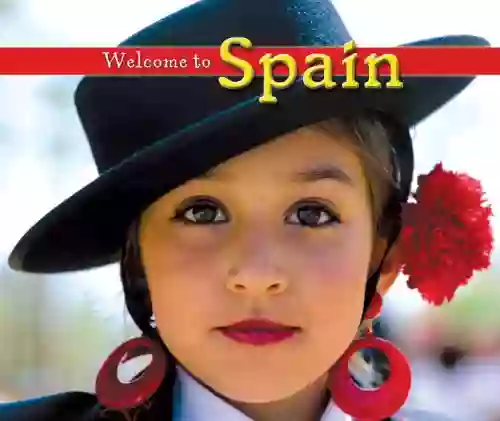

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
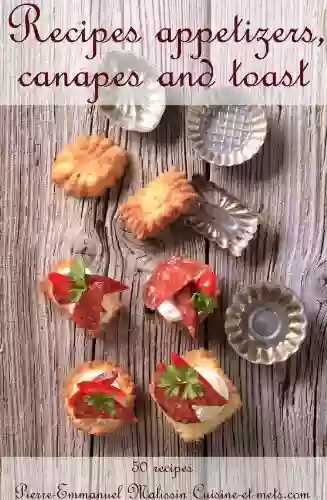

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
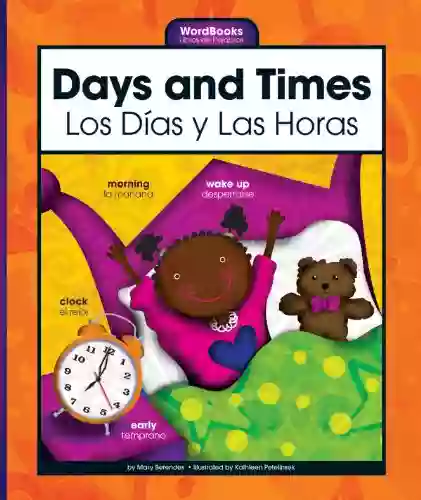

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!
- Elias MitchellFollow ·5.8k
- Brent FosterFollow ·14.4k
- Dave SimmonsFollow ·10.2k
- Roy BellFollow ·7.4k
- Andy ColeFollow ·7k
- Gordon CoxFollow ·14.6k
- Ivan CoxFollow ·15k
- Dan BrownFollow ·4.7k