Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Mastering Arduinojson: Efficient Json Serialization For Embedded Systems

The rise of Internet of Things (IoT) devices has brought about a massive increase in the use of JSON (JavaScript Object Notation) for data exchange between embedded systems. JSON provides a lightweight and human-readable format for structuring data, making it an ideal choice for communication between devices. However, when dealing with resource-constrained embedded systems, efficiency becomes a critical factor to consider.
The Challenge of Serializing JSON on Embedded Systems
Embedded systems often have limited memory and processing power, making it necessary to optimize JSON serialization and deserialization to ensure efficient resource utilization. Arduinojson, a powerful and popular JSON library for embedded systems, has emerged as a go-to solution for addressing these challenges.
Arduinojson is designed with a focus on efficiency and minimal resource usage. It offers an array of features and optimizations that make it exceptionally well-suited for use in IoT and embedded projects. With proper understanding and implementation, developers can master Arduinojson and significantly enhance their embedded systems' JSON serialization performance.
4.7 out of 5
Language | : | English |
File size | : | 13243 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 326 pages |
Understanding Arduinojson's Features and Benefits
Before diving into the intricacies of mastering Arduinojson, it is crucial to understand its key features and benefits that set it apart from other JSON libraries.
1. Static Allocation
Arduinojson allows the allocation of memory for JSON documents at compile-time, eliminating the need for dynamic memory allocation. This feature ensures predictable memory usage and eliminates the risk of memory fragmentation, thereby enhancing the stability and performance of embedded systems.
2. Zero-Copy Deserialization
With zero-copy deserialization, Arduinojson avoids unnecessary memory copying during JSON parsing. By directly accessing the input buffer, the library efficiently parses the JSON data, reducing both memory usage and processing time.
3. Minimal Memory Overhead
Arduinojson strives to minimize memory overhead by avoiding unnecessary memory usage. It offers various options to configure the library's memory footprint, allowing developers to tailor it according to their specific needs.
4. Flexible API
Arduinojson provides a flexible and intuitive API that simplifies the manipulation, creation, and serialization of JSON. It supports various data types, including strings, numbers, arrays, and objects, making it easy to work with complex JSON structures.
Mastering Arduinojson for Efficient JSON Serialization
To achieve efficient JSON serialization on embedded systems using Arduinojson, developers need to follow some best practices and optimization techniques. Let's explore them in detail.
1. Use StaticJsonDocument for Memory Efficiency
Arduinojson offers two types of JSON documents: DynamicJsonDocument and StaticJsonDocument. For most cases, using StaticJsonDocument is recommended as it enables compile-time memory allocation, eliminating the need for dynamic memory management. With a pre-defined buffer size, memory usage is predictable and efficient, ensuring optimal performance.
<pre><code>StaticJsonDocument<256> jsonDocument;</code></pre>
2. Optimize Memory Usage
Arduinojson provides several options to minimize memory usage. For example, by enabling strict mode, the library reduces the memory required to store floating-point numbers. Additionally, disabling features you don't require, such as pretty-printing or support for message pack, can further reduce the memory overhead.
<pre><code>ArduinoJson::enableStrictMode();
ArduinoJson::disableMsgPack();
ArduinoJson::prettyPrintTo(jsonDocument);</code></pre>
3. Use Custom Memory Pool Algorithms
By default, Arduinojson uses the MemoryPool allocator. However, for more granular control over memory allocation, developers can implement custom memory pool algorithms. This allows tailoring memory allocation to the specific requirements of an embedded system, further optimizing memory usage.
4. Minimize String Copies
String manipulation can be memory-intensive, particularly on resource-constrained systems. Arduinojson provides a StringCopier class that allows developers to avoid unnecessary string copies by reusing existing buffers. This optimization technique significantly reduces memory allocation overhead.
5. Enable Streams for Efficient Data Transfer
Arduinojson supports the use of streams for input and output, allowing direct communication with other devices or network interfaces. Streaming data can save memory by processing JSON data in smaller chunks, rather than reading and writing the entire document at once.
<pre><code>ArduinoJson::DeserializationError error = deserializeJson(jsonDocument, Serial);
ArduinoJson::SerializationError err = serializeJson(jsonDocument, Serial);</code></pre>
Mastering Arduinojson is indispensable for developers working on embedded systems and IoT projects requiring efficient JSON serialization. Its unique features and optimizations enable resource-constrained devices to process JSON data reliably and efficiently. By considering the best practices and techniques discussed in this article, developers can improve their understanding of Arduinojson and maximize the performance of their embedded systems.
4.7 out of 5
Language | : | English |
File size | : | 13243 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 326 pages |
Who is this book for?
If you never used ArduinoJson, you will appreciate this book because it guides you through your learning. It starts with the basic usage of the library and increments the complexity step by step. The last chapter analyzes several example projects and explains the design choices. It is a much better way to learn than blindly copy-pasting the examples.
If you are already using ArduinoJson, you will learn how to get the best performance and the most straightforward code. You will discover that many examples found on the Internet are suboptimal and even dangerous. The sample projects will give you a sense of how the library should be used and will give you a new perspective on your projects.
What knowledge is required?
This book is not a course on Arduino or embedded programming. It assumes a small experience with Arduino; you do not need to be a master, just to be able to compile and run a simple sketch.
Many Arduino users lack some of the fundamental principles of C++, that is why this book starts with a quick refresher. However, it does not pretend to teach C++ from scratch; it assumes the knowledge of another object-oriented programming language, such as C# or Java. There is much material to learn how to program with Arduino, but most of them miss essential parts that are crucial in your development career. This chapter covers the holes left by the other Arduino books, that is why I called it "The Missing C++ Course."
What is in the book?
This book is not a compilation of resource already available online. All the content is new, and nothing was recycled from existing documents.
It contains many new code samples and original illustrations.
Contrary to the documentation on arduinojson.org, which grew organically from the GitHub wiki, this book is the result of several months of continuous work, starting from scratch. The result is a coherent and complete learning material.
How is the book written?
This book is optimized for fast and easy reading. It uses short sentences, active voice, and conversational style, to ensure a smooth understanding, even for non-English speakers.
The book is well balanced between text and code. Code snippets are short and focused, so there are easy to digest, but the complete programs are available in the zip file.
The book is designed to be read from cover to cover without a computer, so it is the perfect format to learn in your commute. It is also well segmented, so you can quickly skim when you are looking something in particular.
Why buy this book?
By buying this book, you encourage the development of high-quality libraries. By providing a (modest) source of revenue for open-source developers like me, you ensure that the libraries that you rely on are continuously improved and won't be abandoned after a year.
The current version of the book covers ArduinoJson 5.13, but you will have access to newer revisions as soon as they exist. You only have to buy this book once, you won't have to pay for upgrades.
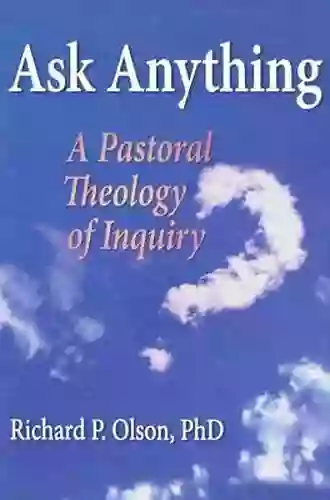

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
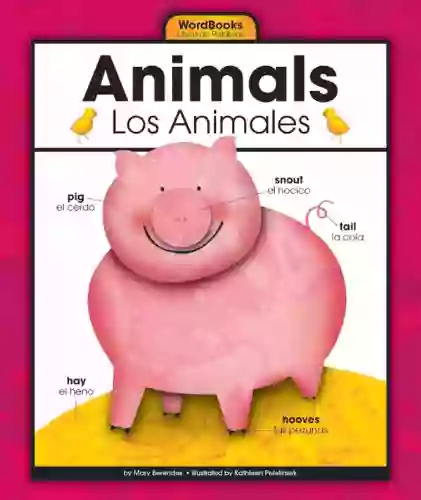

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
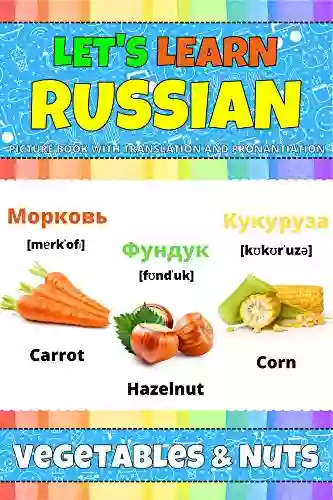

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
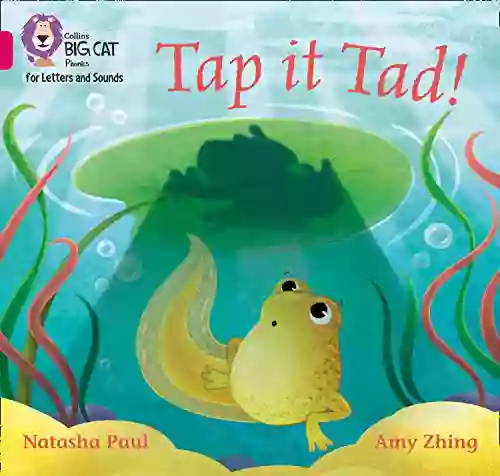

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
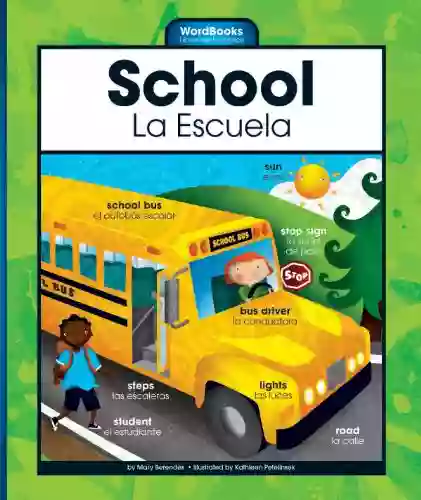

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
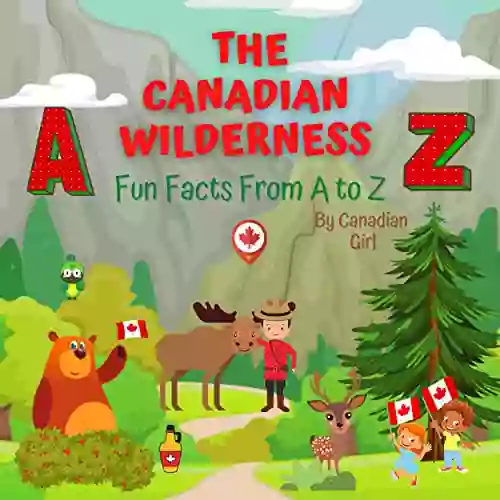

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
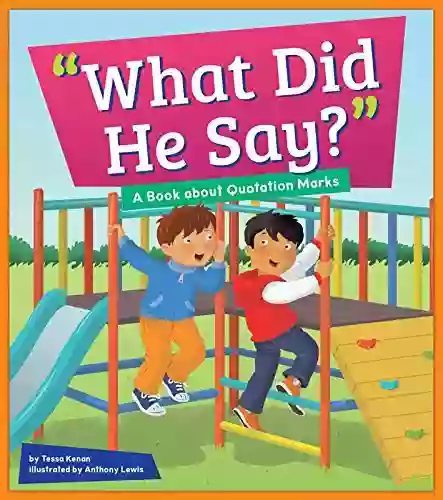

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
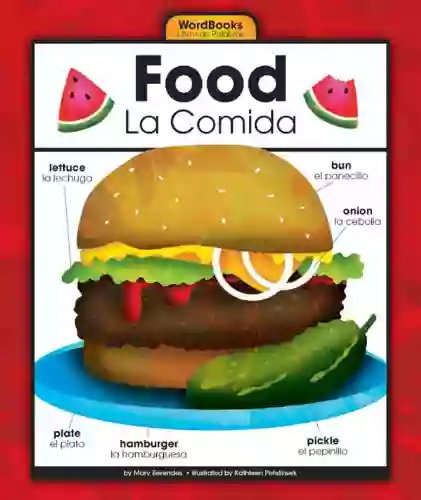

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
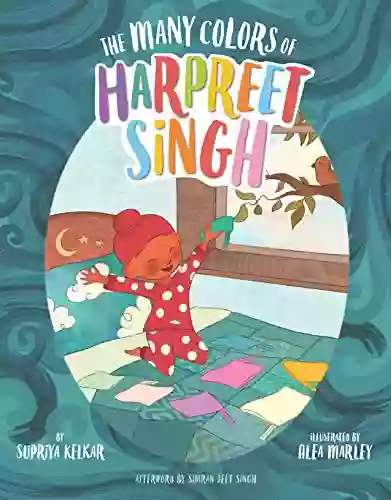

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
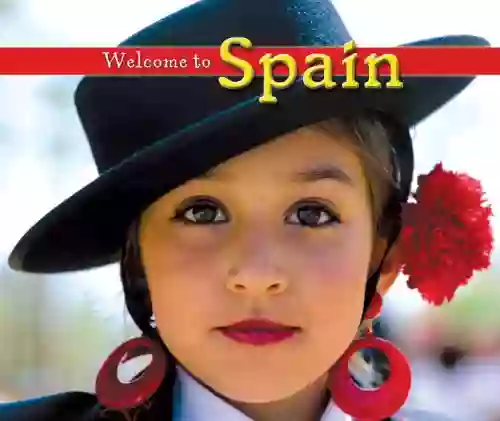

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
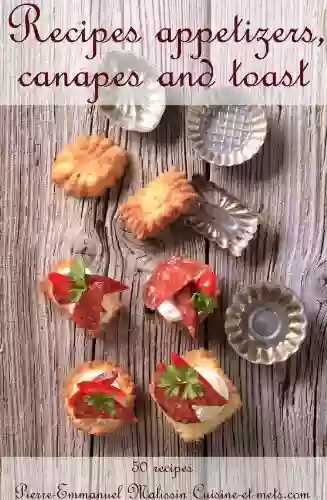

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
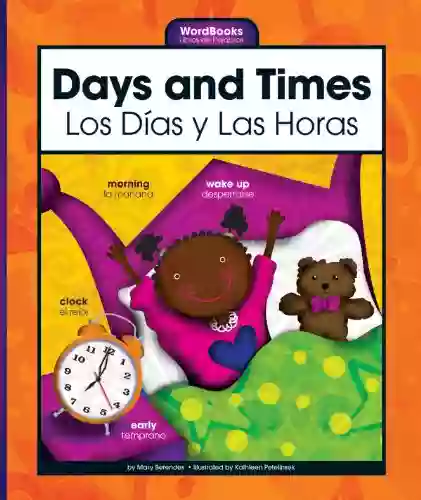

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!




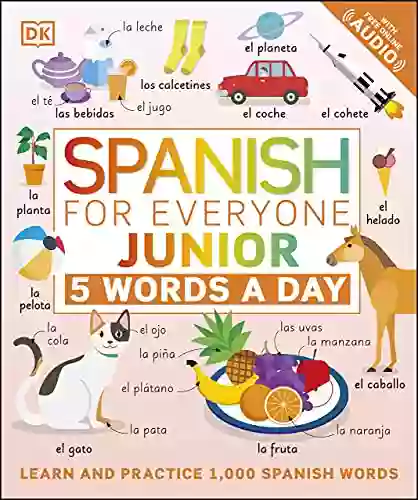

- Roy BellFollow ·7.4k
- Jackson BlairFollow ·12.6k
- Chance FosterFollow ·16k
- Colin RichardsonFollow ·7.7k
- Frank MitchellFollow ·2.5k
- Dale MitchellFollow ·4.7k
- Brian BellFollow ·11.5k
- John ParkerFollow ·17.9k