Do you want to contribute by writing guest posts on this blog?
Please contact us and send us a resume of previous articles that you have written.
Quick Start To Using TypeScript And TypeORM On Node.js For CLI And Web

Are you ready to take your Node.js development to the next level? Do you want to simplify your codebase, make it more maintainable, and reduce bugs? Look no further! TypeScript and TypeORM are here to help!
What is TypeScript?
TypeScript is a statically-typed superset of JavaScript that adds optional static types, classes, and modules to the language. It compiles down to plain JavaScript and is widely used for building large-scale applications. TypeScript enhances the JavaScript development experience by providing syntax checking, autocompletion, and code navigation.
What is TypeORM?
TypeORM is an Object Relational Mapping (ORM) library for TypeScript and JavaScript that simplifies database management. It allows you to interact with databases using JavaScript objects, making it easier to work with data models and relationships.
5 out of 5
Language | : | English |
File size | : | 3258 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 444 pages |
Lending | : | Enabled |
Setting Up TypeScript and TypeORM
To get started, make sure you have Node.js installed on your system. You can check the version by running the following command in your terminal:
node -v
If Node.js is not installed, head over to the official Node.js website and download the latest stable version.
Step 1: Initialize Node.js Project
Create a new directory for your project and navigate to it in your terminal. Then, run the following command to initialize a new Node.js project:
npm init
Step 2: Install TypeScript and TypeORM
Once the project is initialized, install TypeScript and TypeORM as dependencies using the following command:
npm install typescript typeorm
Step 3: Create tsconfig.json File
In the root directory of your project, create a new file named tsconfig.json
and add the following contents to it:
{ "compilerOptions": { "target": "es2020", "module": "commonjs", "esModuleInterop": true, "strict": true, "outDir": "./dist" }, "include": [ "src/**/*.ts" ] }
This configuration file tells TypeScript how to compile your code and where to output the compiled JavaScript files.
Step 4: Create a Simple TypeScript File
In the root directory of your project, create a new directory named src
. Inside the src
directory, create a new file named index.ts
.
Open index.ts
and add the following code:
console.log("Hello, TypeScript!");
Step 5: Transpile TypeScript to JavaScript
Now, run the following command in your terminal to transpile your TypeScript code to JavaScript:
tsc
This command will find all the .ts
files in the src
directory and compile them to JavaScript, placing the output files in the dist
directory.
Step 6: Run the JavaScript File
Finally, you can run the JavaScript file by executing the following command:
node dist/index.js
You should see the message "Hello, TypeScript!
" printed in your terminal, which means everything is working correctly!
Adding TypeORM to Your Project
Now that you have TypeScript set up, let's integrate TypeORM into your project for database management. Follow these steps:
Step 1: Install Database Driver
First, install a database driver that is compatible with TypeORM. For example, if you are using PostgreSQL, run the following command:
npm install pg
You can find a list of supported database drivers on the official TypeORM documentation.
Step 2: Configure Database Connection
In your index.ts
file, import TypeORM and configure the database connection. Here's an example using PostgreSQL:
import { createConnection }from 'typeorm'; createConnection({ type: 'postgres', host: 'localhost', port: 5432, username: 'yourusername', password: 'yourpassword', database: 'yourdatabase', entities: [ ], synchronize: true, logging: true }).then(connection => { console.log("Connected to the database!"); }).catch(error => { console.log("Error connecting to the database:", error); });
Make sure to replace the connection details with your own database credentials.
Step 3: Create Entity Classes
In the same src
directory, create a new directory named entities
. Inside the entities
directory, create a new file named User.ts
and add the following code:
import { Entity, Column, PrimaryGeneratedColumn }from 'typeorm'; @Entity() export class User { @PrimaryGeneratedColumn() id: number; @Column() name: string; @Column() email: string; }
This is a simple example of an entity class representing a user in your database.
Step 4: Run Migrations
Now, you need to run the database migrations to create the necessary tables. In your terminal, run the following command:
typeorm migration:run
This command executes the pending migrations and applies any changes to the database schema.
Step 5: Test the Database Connection
Finally, update your index.ts
file to test the database connection. Add the following code below the database connection configuration:
import { getRepository }from 'typeorm'; getRepository(User).find().then(users => { console.log("Fetched users:", users); }).catch(error => { console.log("Error fetching users:", error); });
This code fetches all users from the database and logs them to the console.
Step 6: Transpile and Run
Transpile your TypeScript code to JavaScript by running tsc
in your terminal. Then, execute node dist/index.js
to run the updated JavaScript file.
Congratulations! You have successfully set up TypeScript and TypeORM on your Node.js project, allowing you to write type-safe code and manage your database with ease.
In this article, we explored how to quickly get started with TypeScript and TypeORM on Node.js for both CLI and web applications. By following the steps outlined above, you can leverage the power of TypeScript's static typing and TypeORM's ORM capabilities to build robust and scalable applications.
Remember to continuously improve your skills and explore the vast ecosystem of libraries and tools available to enhance your development experience with TypeScript and TypeORM.
Happy coding!
5 out of 5
Language | : | English |
File size | : | 3258 KB |
Text-to-Speech | : | Enabled |
Screen Reader | : | Supported |
Enhanced typesetting | : | Enabled |
Print length | : | 444 pages |
Lending | : | Enabled |
Cut to the chase and quickly learn how to use TypeScript and TypeORM in Node.js applications. TypeScript offers a big win to JavaScript programmers developing larger applications. The larger the code base the more important it becomes, in theory, to use static typing and static code analysis techniques to catch certain classes of bugs at compile time.
Long gone are the days when JavaScript was just for snippets on web pages. Today it is used for developing ever larger applications, including Enterprise-scale applications, with large code bases involving dozens or more programmers.
What makes TypeScript compelling is it preserves the best parts of JavaScript while adding enough static type checking to help catch your bugs more quickly. With most IDE's you'll be notified of issues while typing the code.
This book will quickly get you up to speed with using TypeScript to develop Node.js packages and applications (CLI and web).
- Quickly familiarize yourself with both TypeScript and TypeORM in Node.js application development
- Explore developing a Node.js package using TypeScript, then later using it in an Express based web application
- Develop a basic database driven web application, with an advanced ORM layer.
- Explore how TypeScript helps the developer write better code.
- Explore creating TypeORM entities, and all four types of entity relationships in TypeORM
- Explore using decorators to add validation to TypeORM entities
- Use decorators for Express route handlers, and to develop custom Express middleware
- Generate TypeScript type declaration files for regular JavaScript projects
- Convert Node.js/JavaScript projects to TypeScript
The stack used in the example application is:
- Node.js 12.x
- Express, with Bootstrap v5 for the UI
- TypeScript 4.5 and TypeORM 0.2.x
- SQLite3 is used for the database, for simplicity, but TypeORM makes it easy to use many SQL databases and MongoDB
Table of Contents
- to TypeScript
- What is TypeScript? Why is TypeScript so interesting?
- Popularity of TypeScript, JavaScript, Node.js, etc
- What's in this book?
- Quick Start
- Set up Typescript compiler and editing environment
- Implementing NodeJS packages using TypeScript
- Setting up TypeORM, defining the main interface
- Creating TypeORM Entity classes, and CRUD methods
- Letting students register for classes, exploring TypeORM relationships
- Unit Tests for a TypeScript-based CommonJS module
- Validation for values stored with TypeORM Entities
- Building command-line tools (CLI) using TypeScript and OCLIF
- Managing students with the Registrar CLI tool
- Managing classes with the Registrar CLI tool
- Improving the user experience with the cli-ux package
- Configuring Registrar/TypeORM for different databases
- Using Typescript in an Express application
- Setting up the Express server using TypeScript
- Creating the initial Nunjucks templates for an Express application
- Interfacing with back end data storage
- Registrar App User interface - CRUD screens, templates, etc
- Advanced topics in TypeScript on Node.js
- Automating TypeScript development experience
- Making Express handle async router functions
- Improving Express using TypeScript decorators
- Implementing Express middleware with custom TypeScript decorators
- Creating hybrid CJS/ESM modules from TypeScript source
- Complete guide to Entity Relationships in TypeORM
- Using ESLint with TypeScript
- Generating type definition files for regular Node.js JavaScript projects
- Converting regular Node.js/JavaScript packages to TypeScript
- About the author - David Herron
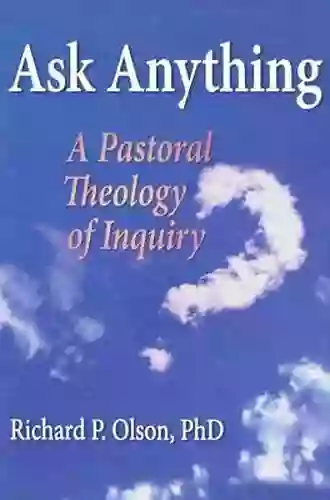

The Secrets of Chaplaincy: Unveiling the Pastoral...
Chaplaincy is a field that encompasses deep...
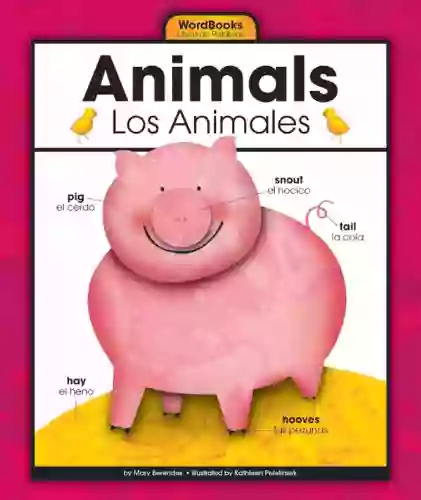

Animales Wordbooks: Libros de Palabras para los Amantes...
Si eres un amante de los animales como yo,...
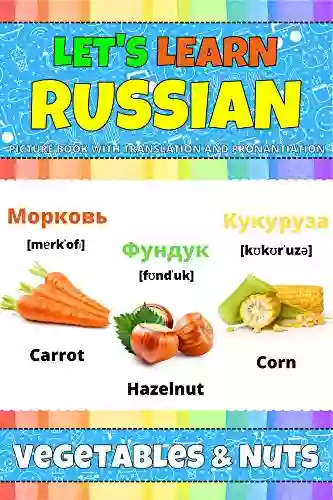

Let's Learn Russian: Unlocking the Mysteries of the...
Are you ready to embark...
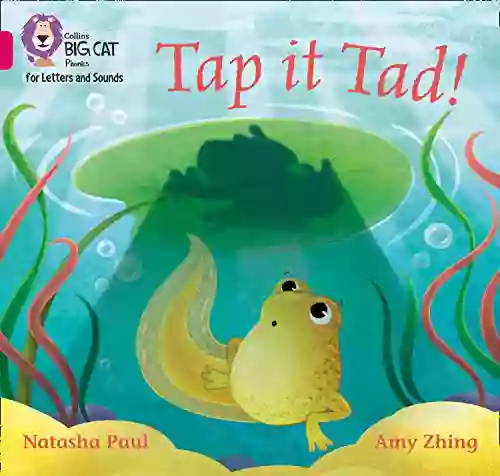

The Incredible Adventures of Tap It Tad: Collins Big Cat...
Welcome to the enchanting world of...
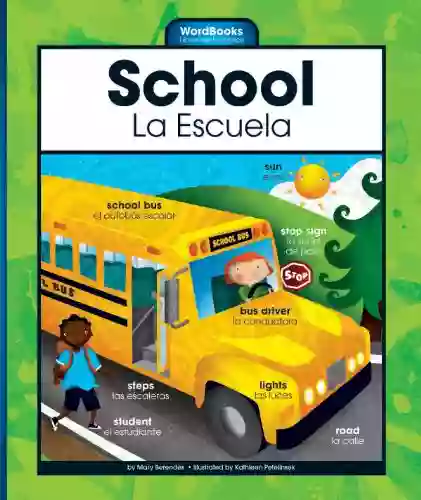

Schoolla Escuela Wordbookslibros De Palabras - Unlocking...
Growing up, one of the most significant...
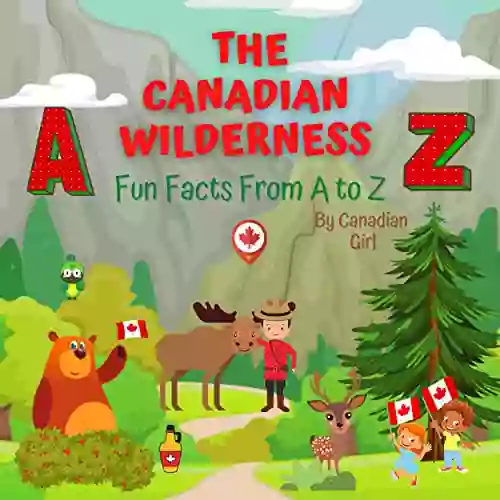

15 Exciting Fun Facts About Canada for Curious Kids
Canada, the second-largest...
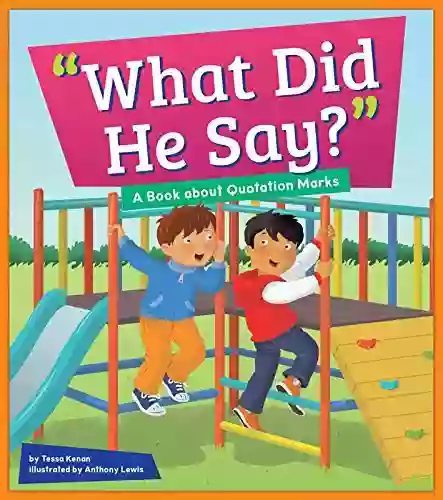

What Did He Say? Unraveling the Mystery Behind His Words
Have you ever found yourself struggling to...
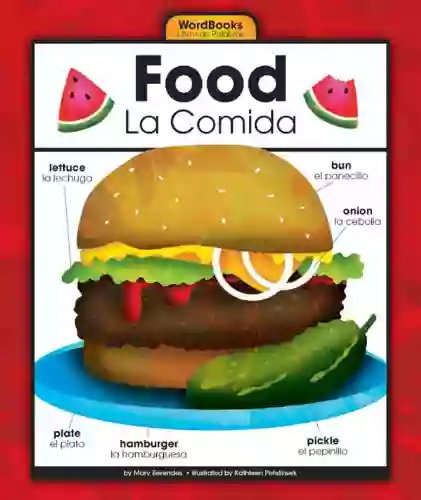

A Delicious Journey through Foodla Comida Wordbookslibros...
Welcome to the world of Foodla Comida...
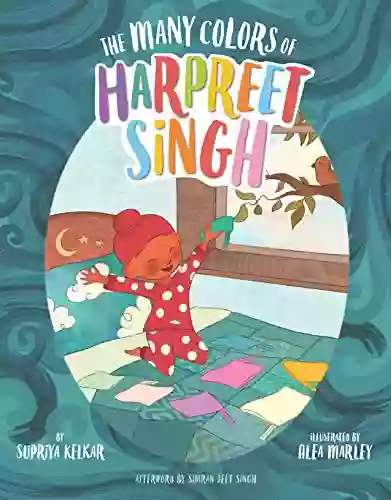

The Many Colors of Harpreet Singh: Embracing...
In a world that often...
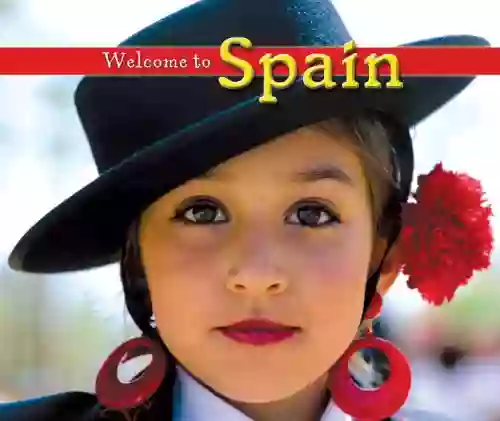

Welcome To Spain Welcome To The World 1259
Welcome to Spain, a country that captivates...
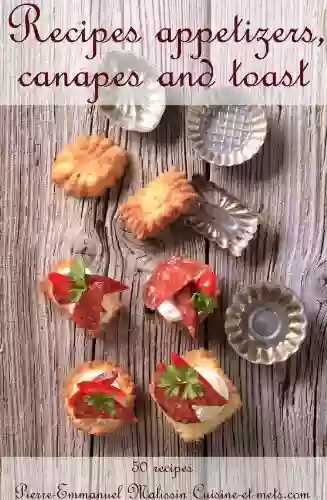

Amazing Recipes for Appetizers, Canapes, and Toast: The...
When it comes to entertaining guests or...
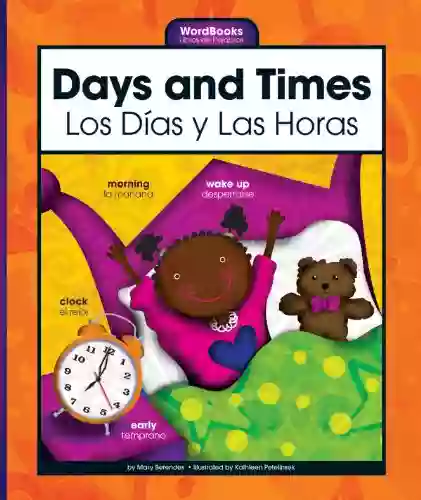

Days And Times Wordbooks: The Ultimate Guide to Mastering...
In the realm of language learning,...
Light bulbAdvertise smarter! Our strategic ad space ensures maximum exposure. Reserve your spot today!




- Ethan GrayFollow ·17.8k
- Henry HayesFollow ·4.9k
- Samuel Taylor ColeridgeFollow ·18.6k
- William GoldingFollow ·8.2k
- Shawn ReedFollow ·15.7k
- Danny SimmonsFollow ·19.1k
- William ShakespeareFollow ·11.9k
- Dan BellFollow ·10.6k